10 Tips for Creating Great Welcome Emails
10 Best Business Email Examples
Reasons Hyper-Localized Marketing Works for SMBs
Ionic Split Pane with Login and Logout System.
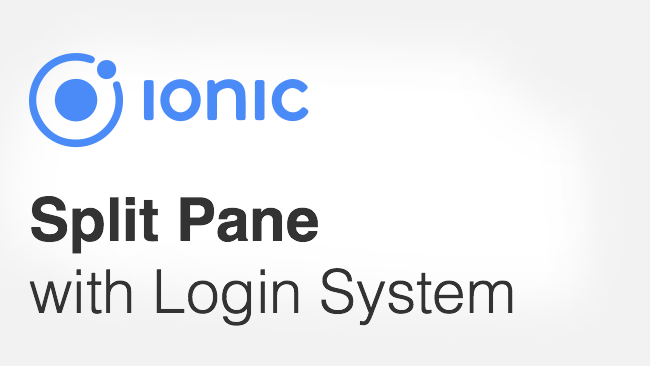
VMagazine: hassle free, powerful WP blog, news & magazine theme
Free Vs Self-Hosted: Which One Is Right For Me?
Rebranding: When Your Company Needs a Fresh Logo to Reinvent Itself Without Losing Individuality
13 Insanely Actionable Free Social Media Tips to Grow Your Business Faster
Who is the Best WordPress Web Hosting Company?
October 2015 Income Report
I wanted to publish an honest income report for this blog… after being inspired by Esteban’s journey
So without further a do here is what I made off of TripleSEO in October.
Thanks
PS Please subscribe to my blog to find out my income in November
PPS Click on the few affiliate links I have on this on this blog and buy some stuff. Christmas is coming and my kids have their mothers spending habits.
Kthxbye
October 2015 Income Report by Chris D – if you enjoyed this post you can read more at TripleSEO or follow Chris on Twitter
Powered by WPeMatico
Implementing Push Notifications: Setting Up & Firebase
You know those the little notification windows that pop up in the top right (Mac) or bottom right (Windows) corner when, for example, a new article on our favorite blog or a new video on YouTube was uploaded? Those are push notifications.
Part of the magic of these notifications is that they can appear even when we're not currently on that website to give us that information (after you've approved it). On mobile devices, where supported, you can even close the browser and still get them.
Article Series:
- Setting Up & Firebase (You are here!)
- The Back End (Coming soon!)

A notification consists of the browser logo so the user knows from which software it comes from, a title, the website URL it was sent from, a short description, and a custom icon.
We are going to explore how to implement push notifications. Since it relies on Service Workers, check out these starting points if you are not familiar with it or the general functionality of the Push API:
What we are going to create

To test out our notifications system, we are going to create a page with:
- a subscribe button
- a form to add posts
- a list of all the previously published posts
A repo on Github with the complete code can be found here and a preview of the project:
And a video of it working:
Gathering all the tools
You are free to choose the back-end system which suits you best. I went with Firebase since it offers a special API which makes implementing a push notification service relatively easy.
We need:
- A Service Worker (and therefore HTTPS)
- A manifest
- Firebase Realtime Database to store our posts
- Firebase Cloud Messaging which lets us "reliably deliver messages at no cost"
- Firebase Cloud Functions which watches changes in our database and sends the notifications
In this part, we'll only focus on the front end, including the Service Worker and manifest, but to use Firebase, you will also need to register and create a new project.
Implementing Subscription Logic
HTML
We have a button to subscribe which gets enabled if 'serviceWorker' in navigator
. Below that, a simple form and a list of posts:
<button id="push-button" disabled>Subscribe</button>
<form action="#">
<input id="input-title">
<label for="input-title">Post Title</label>
<button type="submit" id="add-post">Add Post</button>
</form>
<ul id="list"></ul>
Implementing Firebase
To make use of Firebase, we need to implement some scripts.
<script src="https://www.gstatic.com/firebasejs/4.1.3/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/4.1.3/firebase-database.js"></script>
<script src="https://www.gstatic.com/firebasejs/4.1.3/firebase-messaging.js"></script>
Now we can initialize Firebase using the credentials given under Project Settings → General. The sender ID can be found under Project Settings → Cloud Messaging. The settings are hidden behind the cog icon in the top left corner.
firebase.initializeApp({
apiKey: '<API KEY>',
authDomain: '<PROJECT ID>.firebaseapp.com',
databaseURL: 'https://<PROJECT ID>.firebaseio.com',
projectId: '<PROJECT ID>',
storageBucket: '<PROJECT ID>.appspot.com',
messagingSenderId: '<SENDER ID>'
})
Service Worker Registration
Firebase offers its own service worker setup by creating a file called `firebase-messaging-sw.js` which holds all the functionality to handle push notifications. But usually, you need your Service Worker to do more than just that. So with the useServiceWorker
method we can tell Firebase to use our own `service-worker.js` file as well.
Now we can create a userToken
and a isSubscribed
variable which will be used later on.
const messaging = firebase.messaging(),
database = firebase.database(),
pushBtn = document.getElementById('push-button')
let userToken = null,
isSubscribed = false
window.addEventListener('load', () => {
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('https://cdn.css-tricks.com/service-worker.js')
.then(registration => {
messaging.useServiceWorker(registration)
initializePush()
})
.catch(err => console.log('Service Worker Error', err))
} else {
pushBtn.textContent = 'Push not supported.'
}
})
Initialize Push Setup
Notice the function initializePush()
after the Service Worker registration. It checks if the current user is already subscribed by looking up a token in localStorage
. If there is a token, it changes the button text and saves the token in a variable.
function initializePush() {
userToken = localStorage.getItem('pushToken')
isSubscribed = userToken !== null
updateBtn()
pushBtn.addEventListener('click', () => {
pushBtn.disabled = true
if (isSubscribed) return unsubscribeUser()
return subscribeUser()
})
}
Here we also handle the click event on the subscription button. We disable the button on click to avoid multiple triggers of it.
Update the Subscription Button
To reflect the current subscription state, we need to adjust the button's text and style. We can also check if the user did not allow push notifications when prompted.
function updateBtn() {
if (Notification.permission === 'denied') {
pushBtn.textContent = 'Subscription blocked'
return
}
pushBtn.textContent = isSubscribed ? 'Unsubscribe' : 'Subscribe'
pushBtn.disabled = false
}
Subscribe User
Let's say the user visits us for the first time in a modern browser, so he is not yet subscribed. Plus, Service Workers and Push API are supported. When he clicks the button, the subscribeUser()
function is fired.
function subscribeUser() {
messaging.requestPermission()
.then(() => messaging.getToken())
.then(token => {
updateSubscriptionOnServer(token)
isSubscribed = true
userToken = token
localStorage.setItem('pushToken', token)
updateBtn()
})
.catch(err => console.log('Denied', err))
}
Here we ask permission to send push notifications to the user by writing messaging.requestPermission()
.

If the user blocks this request, the button is adjusted the way we implemented it in the updateBtn()
function. If the user allows this request, a new token is generated, saved in a variable as well as in localStorage
. The token is being saved in our database by updateSubscriptionOnServer()
.
Save Subscription in our Database
If the user was already subscribed, we target the right database reference where we saved the tokens (in this case device_ids
), look for the token the user already has provided before, and remove it.
Otherwise, we want to save the token. With .once('value')
, we receive the key values and can check if the token is already there. This serves as second protection to the lookup in localStorage
in initializePush()
since the token might get deleted from there due to various reasons. We don't want the user to receive multiple notifications with the same content.
function updateSubscriptionOnServer(token) {
if (isSubscribed) {
return database.ref('device_ids')
.equalTo(token)
.on('child_added', snapshot => snapshot.ref.remove())
}
database.ref('device_ids').once('value')
.then(snapshots => {
let deviceExists = false
snapshots.forEach(childSnapshot => {
if (childSnapshot.val() === token) {
deviceExists = true
return console.log('Device already registered.');
}
})
if (!deviceExists) {
console.log('Device subscribed');
return database.ref('device_ids').push(token)
}
})
}
Unsubscribe User
If the user clicks the button after subscribing again, their token gets deleted. We reset our userToken
and isSubscribed
variables as well as remove the token from localStorage
and update our button again.
function unsubscribeUser() {
messaging.deleteToken(userToken)
.then(() => {
updateSubscriptionOnServer(userToken)
isSubscribed = false
userToken = null
localStorage.removeItem('pushToken')
updateBtn()
})
.catch(err => console.log('Error unsubscribing', err))
}
To let the Service Worker know we use Firebase, we import the scripts into `service-worker.js` before anything else.
importScripts('https://www.gstatic.com/firebasejs/4.1.3/firebase-app.js')
importScripts('https://www.gstatic.com/firebasejs/4.1.3/firebase-database.js')
importScripts('https://www.gstatic.com/firebasejs/4.1.3/firebase-messaging.js')
We need to initialize Firebase again since the Service Worker cannot access the data inside our `main.js` file.
firebase.initializeApp({
apiKey: "<API KEY>",
authDomain: "<PROJECT ID>.firebaseapp.com",
databaseURL: "https://<PROJECT ID>.firebaseio.com",
projectId: "<PROJECT ID>",
storageBucket: "<PROJECT ID>.appspot.com",
messagingSenderId: "<SENDER ID>"
})
Below that we add all events around handling the notification window. In this example, we close the notification and open a website after clicking on it.
self.addEventListener('notificationclick', event => {
event.notification.close()
event.waitUntil(
self.clients.openWindow('https://artofmyself.com')
)
})
Another example would be synchronizing data in the background. Read Google's article about that.
Show Messages when on Site
When we are subscribed to notifications of new posts but are already visiting the blog at the same moment a new post is published, we don't receive a notification.
A way to solve this is by showing a different kind of message on the site itself like a little snackbar at the bottom.

To intercept the payload of the message, we call the onMessage
method on Firebase Messaging.
The styling in this example uses Material Design Lite.
<div id="snackbar" class="mdl-js-snackbar mdl-snackbar">
<div class="mdl-snackbar__text"></div>
<button class="mdl-snackbar__action" type="button"></button>
</div>
import 'material-design-lite'
messaging.onMessage(payload => {
const snackbarContainer = document.querySelector('#snackbar')
let data = {
message: payload.notification.title,
timeout: 5000,
actionHandler() {
location.reload()
},
actionText: 'Reload'
}
snackbarContainer.MaterialSnackbar.showSnackbar(data)
})
Adding a Manifest
The last step for this part of the series is adding the Google Cloud Messaging Sender ID to the `manifest.json` file. This ID makes sure Firebase is allowed to send messages to our app. If you don't already have a manifest, create one and add the following. Do not change the value.
{
"gcm_sender_id": "103953800507"
}
Now we are all set up on the front end. What's left is creating our actual database and the functions to watch database changes in the next article.
Article Series:
- Setting Up & Firebase (You are here!)
- The Back End (Coming soon!)
Implementing Push Notifications: Setting Up & Firebase is a post from CSS-Tricks
Don’t Fall Into This Trap That Could Destroy Your Blog
Last week I spent time with a young blogger who was completely stalled with her blog (for the purpose of this post I’ll call her Sally).
Sally’s blogging had started with a bang and had put together 3 great months of content and had started to build a readership but then it suddenly all came to a halt.
I arranged to catch up for coffee to see what had happened and see if there was a way to get her moving again and she told me a story that I’m sure many readers will find familiar.
Paralysed by Comparisons
The reason Sally started blogging was that she had been a reader of another reasonably well known blogger. She had been so inspired by this established blogger that she simply had to start her own blog – which she did.
The problem that brought Sally’s blog to a grinding halt started a few weeks after her blog began when Sally began to compare her fledgling blog with her hero’s blog.
It started innocently enough with her noticing that this others blogger’s design just seemed to flow much better than Sally’s. However in the coming days and weeks Sally started to compare other things too.
Her hero seemed to blog with more confidence, she got more comments, she had a larger Twitter following, she was more active on Pinterest, she was getting some great brands advertise on her blog, she was invited to cool events…
Once Sally started comparing she couldn’t stop. She told me that she would sit down to work on her blog and end up on her hero’s blog and social media accounts – for hours on end – comparing what they were doing.
On one hand Sally knew it wasn’t a fair comparison – she had only been blogging by this stage for a couple of months and her hero had been blogging for over 4 years… but logic was clouded out by jealousy and Sally found her blogging beginning to stall.
She started second guessing herself. She would work for days on blog posts – hoping to perfect them to the standard of her hero only to get to the point of publishing them and trashing them instead for fear of them not being up to scratch.
Days would go by between posts and then weeks. Sally’s blog began to stall… and then it died completely.
The Comparison Trap
Sally isn’t the only blogger to fall into the trap of comparing oneself with others – in fact I’ve heard this story (or variations of it) numerous times. If I’m honest, it’s something that at times I’ve struggled with too.
I remember in the early days of my own blogging comparing my style of writing with other bloggers that I admired who wrote in a much more academic, heavy style of writing. I tried to emulate this over and over again and never felt I hit the benchmark that they set.
The temptation was to give up – but luckily I found my more informal and conversational voice through experimentation and persistance.
Comparing Is Never Fair
As I chatted with Sally last week a theme emerged in our conversation – the comparisons were simply not fair.
Sally knew this on some levels but needed to hear it again.
Her hero had been blogging for years. Sally had been blogging for months.
Not only that – Sally was comparing herself to tiny snapshots of this other blogger.
She could see her hero’s Twitter follower numbers, how many comments she was getting, how many times she Pinned on Pinterest and the instagram photos of this blogger at glamorous events – but she didn’t really have the full picture of this other blogger.
She didn’t know how many hours that blogger worked, she didn’t know whether that other blogger had people working for her, she didn’t know if that other blogger was actually happy with her blog or life and she certainly didn’t see the instagrams of that other bloggers boring, dull or hard moments of life.
I’m not saying the other blogger is hiding anything or doing anything wrong – just that the comparisons Sally was making were of everything Sally knew about herself (and her insecurities) with tiny edited snapshots of the life and work another person.
Run You Own Race
Sally is a remarkable person. I’d love to tell you her real name and story because she’s overcome some amazing things in her life, has some unique perspectives to share and has an inspirational story to tell.
My encouragement to Sally (and to us all) is run her own race. Yes she’s running beside others that at times seem to be running faster or with more flare… but nobody else around her has her unique personality, set of experiences or skills.
Nobody else can blog like Sally – so the sooner she gets comfy in her own skin the better.
Don’t Fall Into This Trap That Could Destroy Your Blog
http://www.problogger.net/archives/2013/09/04/dont-fall-into-this-trap-that-could-destroy-your-blog/
http://www.problogger.net/archives/category/miscellaneous-blog-tips/feed/
@ProBlogger» Miscellaneous Blog Tips
Blog Tips to Help You Make Money Blogging – ProBlogger
http://www.problogger.net/wp-content/plugins/podpress/images/powered_by_podpress_large.jpg
5 Intellectual Property Laws about the Internet Bloggers Need to Know
This is a guest contribution from JT Ripton, a Freelance writer from Tampa.
Intellectual property law protects much of the content that you enjoy on the internet. Though you aren’t always required to pay to enjoy this content, that doesn’t make it free for all types of use. Since many intellectual property laws haven’t yet been adapted specifically for the Internet, here’s a rundown of the basics you can use to safely guide your decisions.
Photos and Images are Not Free for Use
Quick and simple searches like Google Images make it seem like the Internet is overflowing with free photos and images. However, copyright law protects most of these photos. If you’re looking for images you can post on your blog, you need to look for those with a Creative Commons license. You can also pay for rights to use certain images.
Creative Commons Licenses Come in Different Forms
Creative Common licenses give you access to various forms of intellectual property. There are many different types of Creative Commons licenses. Before using something that’s protected under this type of license, you must carefully look at it to decide exactly how you can use the image. Some licenses allow commercial use while others do not. Some allow you to alter an image while others stipulate that it must stay in its original form. Attribution is typically required.
Most Movies, Music, and Television are Protected
Although there are many sites where you can get access to movies, television shows, and music for free, these downloads are typically illegal. Though the sites themselves are not violating any laws, you are if you share or download copyrighted material. You can legally view some movies and shows online, but you cannot download them. Network sites often show recent episodes of popular shows and sites like Netflix and Hulu offer access to movies and shows with a paid subscription.
Plagiarism Isn’t Just for School Papers
You undoubtedly learned about the dangers of plagiarism in high school and college, but these laws’ importance doesn’t end when you’re finished writing term papers and dissertations. Whether you have a blog yourself or you write for others, you cannot reproduce another’s intellectual property and take credit for it as your own. Cite your sources, use quotation marks when needed, and try to limit your works to your own unique ideas as much as possible. Referencing another article and quoting from a book are fine. Reposting an entire article or chapter of a published piece are not.
Permission Trumps All
When in doubt about a work, simply ask for permission to use it. Just as the Internet make it easy to find works, so too does it make it easy to contact creators. Many will gladly give you the necessary permissions when requested.
The Internet is a great forum for sharing everything from thoughts and ideas to your original photos, films, and musical works. However, it’s essential that you always think about who has the rights to the content in question and act so that you do not violate them.
JT Ripton is a Freelance writer from Tampa, FL, he writes about several of his interests including, blogging, all things tech, and useful tips and idea’s for a myriad of things. He likes to write to inform and intrigue.
5 Intellectual Property Laws about the Internet Bloggers Need to Know
http://www.problogger.net/archives/2013/08/16/5-intellectual-property-laws-about-the-internet-bloggers-need-to-know/
http://www.problogger.net/archives/category/miscellaneous-blog-tips/feed/
@ProBlogger» Miscellaneous Blog Tips
Blog Tips to Help You Make Money Blogging – ProBlogger
http://www.problogger.net/wp-content/plugins/podpress/images/powered_by_podpress_large.jpg
Set Your Blog for Success With These Simple Tactics
This is a guest contribution from freelance writer, Ayelet Weisz.
Blogging is hard work.
You need to come up with fresh, quality materials on a regular basis, promote them, connect with readers, network with peers and mentors – and that’s before you even see a single dollar for your effort.
I’ve put together some simple business tactics to help you set your blog for success, so you can live the pro blogger dream.
Set Inspiring (But Realistic) Goals
Now that you’re your own boss, you’ve got to set up internal motivation. The biggest success stories didn’t get there with someone telling them what to do every five minutes.
A great way to keep yourself motivated is to set up goals. Of course, I don’t just mean any goal. Making a million dollars by the end of your first year as a blogger might not be the most realistic goal you could think of.
The truth is, you have no way of knowing what will happen by the end of your first year, and you have no control over of others’ choices – Will they read your blog? Will they buy your products?
However, you can eliminate some of these unknown factors by conducting research about the possibilities your market contains – and you can increase the chances of realising your dreams by setting a different type of goal.
Focus on what kind of content you’ll write, how much content you’ll write, how you’ll promote it and when.
Focus on numeral items, like 8 posts a months on your blog and 8 guest posts that you’ll pitch to big blogs. Don’t set a goal of publishing 8 guest posts, only of submission. If someone says no, you’ll still have the confidence boost of reaching your goal. Then, you can exceed it by pitching that guest post to an additional blog.
Track Your Progress
The next step is to get the gold stars out track your progress. Write down what you are doing, what your productivity rate has been and notice what times a day or situations support you in getting more work done.
Set a meeting with yourself – be it once a week, once a month or once a quarter – to see how well you did, to discover your strong points. It’s important to be honest on where you need to be more accountable or get support.
Encourage yourself to ask questions, to say “I don’t know”, to ask for help. Sometimes, that help will come in the form of adjusting your expectations or re-shaping your schedule. Embrace your humanity as you embrace your new blogging journey.
Give yourself time, be gentle – and leverage your failures
Starting a new venture is never easy. Acquiring an abundance of new skills and tools takes determination, focus and accountability.
You will make mistakes.
Give yourself a time of grace and don’t be hard on yourself. People around you might pressure you. They could be your friends and family members, they could love you and want the best for you – and they might not believe your blog is what’s best for you. If it takes you time to monetize your blog, and it probably will, they’ll doubt it even more.
Don’t get carried away with that. It will take time. Embrace it as an opportunity to show yourself you can do the impossible.
Support yourself through this time. Join professional online and offline groups, share your challenges with people who understand rather than with people who don’t, and plan ahead financially.
It might work best for you to save a few months’ or a year’s worth of salary, then take that time off paid employment and market like there’s no tomorrow. Alternatively, it might be best for you to start building your blog slowly, as you keep a part time or full time job.
Expect to make mistakes.
These mistakes will be your guiding points to grow your blog even more as you go on. They could be transformed into guest posts on big blogs, case studies you can use to show your expertise (and how you turned failure to success) – and they can even turn you into a good mentor one day!
If nothing else, you’ll be able to look back one day and have a really good laugh. You’ll also be able to see how far you’ve come.
Socialise
Starting out at the blogsphere can be intimidating.People already know each other and the job.Friendships and communities have already been formed. Relationships with influencers are being shaped and re-shaped every single day.
This experience becomes easier once you feel there’s someone you could turn to. You, of course, need to have communication tools and the courage to connect when entering a new environment.
If you’re fearful about connecting with industry members, start small. Post comments on their blogs, then connect with them on Twitter or Facebook. Join online communities and reach out to one person at a time in a personal message.
Ask for their help, or offer a solution to a challenge they brought up. If they happen to just start out as you are, perhaps you could be a force of empowering support to one another, sharing tips and encouraging each other when one loses sight of the light at the end of the tunnel.
Maybe you’ll even find you have additional interests in common!
Did you face any of these challenges when you were starting out? How did build your blog? Or perhaps you’re just starting out and picked up some great tips for the road ahead. Share your story!
Ayelet Weisz (www.AyeletWeisz.com) is an enthusiastic freelance writer, blogger and screenwriter, who focuses on business, technology, travel and women’s issues. Get her free report, 48 Must-Live Israeli Experiences, on her travel blog, and connect with her on Twitter.
Set Your Blog for Success With These Simple Tactics
http://www.problogger.net/archives/2013/06/11/set-your-blog-for-success-with-these-simple-tactics/
http://www.problogger.net/archives/category/miscellaneous-blog-tips/feed/
@ProBlogger» Miscellaneous Blog Tips
Blog Tips to Help You Make Money Blogging – ProBlogger
http://www.problogger.net/wp-content/plugins/podpress/images/powered_by_podpress_large.jpg
3 Ways to Get More Subscribers for Your Blog
“I have hundreds of readers coming to my blog every day – but nobody ever subscribes to my newsletter. Help!?!”
This request came in via email today and I thought I’d share my reply with the 3 suggestions I offered.
—–
Thanks for the question – I suspect you’re not alone with this problem. While a lot can probably be written on the topic, let me suggest 3 things I’ve found helpful increasing subscriber numbers.
Note: the #1 thing I did to building subscriber numbers on Digital Photography School was introduce a lightbox subscriber box. I spoke about this in my 10 Things I Wish I Knew About Blogging webinar so I won’t rehash it here.
1. Ask People to Subscribe
This sounds a little too simple to be effective but I’m amazed how many people do subscribe once you mention you’ve got a newsletter. I’m also amazed how many of our regular and loyal readers don’t know we even have a newsletter, despite it being promoted around the blog.
Some semi-regular calls to subscribe can be very effective.
You can do this in a number of ways, including:
- Writing a dedicated blog post, every now and again, explaining you have a newsletter and the benefits of subscribing.
- Mentioning your newsletter in passing in your blog posts. I don’t mean every single post, but a mention now and then will work wonders.
- Promoting your newsletter across social media. I regularly mention our newsletters on Twitter, when I’m writing a newsletter and when it goes out.
The key to remember, when mentioning your newsletter regularly, is to find fresh ways to talk about it. Don’t just have the same tweet to subscribe every 2nd day.
- Mention something that is in the next newsletter, that you won’t get anywhere else.
- Mention that you’ve just hit a milestone number of subscribers to build social proof.
- Mention that it’s a milestone newsletter. We recently sent out 250th on dPS and made a bit of a big deal about it.
2. Start a Series
Announce that you’re going to be doing a series of posts, on your blog, on a topic that you know will really be useful to your readers.
I remember the first time I announced that I was going to run the 31 Days to Build a Better Blog series (the series that later became the eBook by the same name) I was amazed at how many people subscribed to my blog over the next 24 hours.
I was signalling to readers that I was going to do something that would serve them and in doing so, created anticipation among my readers. This anticipation (as I’ve written about in the past) is a key reason people will subscribe to your blog.
3. Place Calls to Subscribe in ‘Hot Zones’
One last tip is to identify some ‘hot zones’ on your blog, to place calls to subscribe. These zones are either places that your readers will be looking or pages that they’ll be visiting.
Let me suggest a couple:
1. Under Posts
I’m not currently doing this on my blogs, as I use the space under my blog posts for other things, but I’ve found over the years that the area under your blog post (and directly above comments) is a ‘hot zone’ where readers often look for what to do next.
Put yourself in the position of a reader. You’ve read the post and have found it useful. This is the perfect time to ask readers to subscribe because they’re hopefully feeling satisfied, stimulated and helped in some way.
A bold call to subscribe can work wonders here.
2. On Hot Posts
Dig into your blogs analytics package and identify which posts are the most read posts on your blog.
You’ll probably find that these posts are receiving traffic from search engines and are likely being read by first time readers to your blog – people that are often quick to leave again once they’ve got what they’re searching for.
These posts are a real opportunity to make your blog a little more sticky and to hopefully call some of those first time readers to subscribe.
You can do this either by adding a call to subscribe directly to the posts – or you might like to link from these posts to a ‘sneeze page’ (see below).
3. Sneeze Pages
Let me show you a page on dPS, which is a page that generates a large number of subscribers. It is our Photography Tips for Beginners page.
This page is a page in which I link to 33 of the best articles in our archives for beginner photographers. It is a page that ‘sneezes’ readers deep into our archives to good quality content.
It is a great page for driving traffic and getting readers deep into the site but you’ll also note we have a couple of strong calls to subscribe on that page. People click those calls to action like crazy because they can see on the page that we’ve created a heap of useful content.
We link to this sneeze page prominently in the navigation areas all around the site to drive traffic to it and regularly promote the page on social media (as I write this it has received over 90,000 ‘pins’ on Pinterest for example).
Take home lesson – create a sneeze page with a strong call to action to subscribe and drive as much traffic to it as you can!
Note: Sneeze pages are written about on day 18 of 31 Days to Build a Better Blog.
How Have You Increases Subscribers to Your Blog?
I have barely scratched the surface here on how to increase subscribers to a blog and would love to hear your suggestions and experiences on the topic in comments below.
What has worked for you?
3 Ways to Get More Subscribers for Your Blog
http://www.problogger.net/archives/2013/06/21/3-ways-to-get-more-subscribers-for-your-blog/
http://www.problogger.net/archives/category/miscellaneous-blog-tips/feed/
@ProBlogger» Miscellaneous Blog Tips
Blog Tips to Help You Make Money Blogging – ProBlogger
http://www.problogger.net/wp-content/plugins/podpress/images/powered_by_podpress_large.jpg
Increase your SEO with Team Content Marketing!
This is a guest blog contribution from Matt Ganzak, founder of EliteGurus.com and BuildNetworkPlus.com.
Content marketing and SEO is getting more difficult. Each day, there are thousands of new domains purchased and thousands of new websites going online.
Most new bloggers and content writers will get started with their site, staying active for a couple months, and then reality hits them. No traffic to their articles. The time does not seem worth the effort.
Content Marketing can be FRUSTRATING!
In an effort to remove outdated and irrelevant search results, Google has been updating their systems over the years with a series of updates. The latest Google update, Penguin, was said to lower the ranks for websites that have poor quality links and also took into consideration the relevancy.
This was the push some businesses and bloggers needed to find a better way to build quality SEO.
Today’s Content Marketing Strategy for SEO
According to Google, the best way to get quality rankings is to follow this guide:
1) Post quality content that gets shared
2) Have social widgets easy to access
3) Use Google Plus profiles for authors
4) Use Google Plus Pages for sites
5) Guest blog on other sites to earn link backs
6) Do not buy links
7) Setup your web pages targeting keywords
8) But do not over optimize
In a nutshell, there is no shortcut to boosting your organic traffic, so just put out the quality content and they will come. Well, this strategy is causing newbie bloggers to get frustrated.
So what is the solution?
I have been teaching my clients to build networks within their niche industry, and share each other’s articles. This is a strategy that will build your organic SEO naturally as all the sites work together to grow traffic. Many bloggers choose to be loners and just focus on “their audience” but realistically, today’s Google Algorithm stacks the cards against these loners.
Fact is, you need to reach out and make new friends. Connect on the social networks, and reshare each other’s content. Then also guest blog on each other’s sites and even send newsletter’s to each other’s lists. If you build small niche teams and work together, Google will build your PageRank and Domain Authority as your sites grow together.
This strategy does not create overnight success, and can be rather time consuming. But Google will index their community with excellent authority and continue to do so as her network grows.
Steps to creating your Network
The first step is to decide on your niche market, and do not stray from it! If you confuse Google by having so many different industries, Google will not index you with high relevance for your target industry. Choose and stay the course.
Next, determine who your competitors are in your vertical. You can do this with a Google search for your keywords. And I always like to check Alexa.com rankings to get a look at their traffic score.
After that, choose relevant keywords with high search volume but low competition. I suggest picking 4-7 keywords to target. If you choose more, you can spread your site too thin which will lower your keyword density for your target keywords.
Lastly, find out where your niche industry peers hang out. This can literally mean go to networking events in person, or meetup groups. Take business cards! But you will also want to find online communities dedicated to your niche. Get in, get involved and start networking together.
Personally, I have been doing this sort of networking for the past year and my group has been growing. If you would like to join, I would be more than happy to chat and put up guest posts on any of my websites.
So let’s all work together to improve our PageRank and Domain Authority to grow our readership together. Content Marketing and SEO has become a team effort. I look forward to meeting each and everyone one of you.
Matt has been working in marketing for the past 12 years. He is an innovator of new ideas and has been training businesses on building their online presence. He specializes in Website Development, SEO, blogging, PPC, media buying and monetization strategy. Connect with Matt on EliteGurus.com, and be sure to check out his WordPress hosting (DollarWebsiteClub.com).
Increase your SEO with Team Content Marketing!
http://www.problogger.net/archives/2013/08/31/increase-seo-with-team-content-marketing-approach/
http://www.problogger.net/archives/category/search-engine-optimization/feed/
@ProBlogger» Search Engine Optimization
Blog Tips to Help You Make Money Blogging – ProBlogger
http://www.problogger.net/wp-content/plugins/podpress/images/powered_by_podpress_large.jpg
How I Stopped Waiting to Become a Writer, Quit My Job & Launched My Dream
This is a guest contribution from Jeff Goins of Goins, Writer.
It took me six years to become a professional blogger. And four and a half of those years were spent waiting.
For years, I read blogs, bought books, and watched videos about ordinary, everyday people making the colossal shift from day job to living their dream. I seethed with envy and bitterness as I saw friends skyrocket to success, living out their passions.
What were they doing that I wasn’t?
All the while, I waited. Waited for someone to pick me. Waited for permission. Waited to be good enough to start.
And guess what? Permission never came. Until one day, when everything changed…
The Conversation That Turned Me into a Writer
A few years ago, a friend asked me an important question:
“What’s your dream?”
“Don’t have one,” I said.
“Sure you do. Everyone has a dream.”
“Ah, I dunno… I’m living it, I guess.”
“Really? Hrmph.” And then a long pause — “Because, well, I would’ve thought your dream was to be a writer.”
As soon as I heard those words, something in me stirred. Something that had been there all along.
“Well, uh, yeah…” I gulped, “I guess I’d like to be a writer… some day. But that’ll never happen.”
I sounded so sure, so certain that at 28 years old, I knew where the rest of my life was headed. Shaking his head, my friend smiled.
“Jeff… You don’t have to want to be a writer…”
And then he said nine words that changed me life:
“You are a writer; you just need to write.”
Turns out that was all I needed. It’s really all any of us need: to believe we already are what we want to be.
So that’s what I did. I started calling myself a writer. And I started practicing.
Practice Makes Better
After that conversation, I started blogging, guest posting (despite my fear of rejection), and sharing my work with the world. At first, nobody noticed or cared, and that was just fine with me. Because I was finally doing what I was born to do: writing.
It was good to blog in relative obscurity, good to practice without the whole world watching. This is a foreign concept in our world today. Everyone wants to be awesome now, but the road that leads to mastery is rarely a densely-populated one.
At the same time, I had a daily routine. I was, as Seth Godin says, practicing in public. Putting myself on the line. Forcing myself to be creative. Every day by 7:00 a.m., I had to post something. Seven days a week, 365 days a year. And this expectation I placed on myself was just what I needed. It made me better, helped me find my voice.
That one year of intense writing taught me more than the previous six years of writing when I felt like it. The lesson I learned was this: frequency, not quantity, is what counts in getting to excellence. Some days, I wrote for 30 minutes, while others I wrote for two hours. But the amount of time didn’t matter.
What mattered was that I showed up.
And that’s what turned a hobby into a discipline — and eventually a profession.
“This is the… secret that real artists know and wannabe writers don’t. When we sit down each day and do our work, power concentrates around us. The Muse takes note of our dedication. She approves. We have earned favor in her sight.” —Steven Pressfield
How I Built an Audience of 100,000 Readers in 18 Months
Initially, nobody knew me. So I interviewed influential people and A-list bloggers, people I wanted to learn from and associate with. And after awhile, I became friends with these folks, some of which invited me to write on their blogs.
Still, there were no overnight successes. It took me six months to get a mere 75 email subscribers. But something amazing happened around that six-month mark: The week I released a free, 900-word eBook for writers, I had over 1000 signups in seven days.
There was power, I learned, in giving more than taking, being generous instead of greedy.
Eight months in, I had amassed an audience of a few thousand followers (through guest posting and my free eBook), and a book publisher asked me to write a book. Within 18 months, those 1000 emails had turned in 20,000, and I was regularly receiving over 100,000 unique visitors to my blog each month.
I wasn’t making much money yet, but I believed that if I helped people, there would be a way to make a living.
From Side Project to Full-time Income
That next year, my wife and I were expecting our first child, and we weren’t sure how we were going to afford it. She wanted to stay home and raise our son, and on my salary it just wasn’t possible.
Someone told me that once you had over 10,000 subscribers, you had a six-figure business. So I decided to give that idea a try, throwing together an eBook in a few days. I sent an email to my subscribers, telling them I was offering the eBook at an early discounted rate. With that first eBook, I made about $1500 in a weekend. At the time, that was about half a month’s salary for me, an entire paycheck. In two days.
I couldn’t believe it. The next few months, I played around with affiliate products and started making a couple hundred bucks a month doing that. The side income was nice, but I knew I had to do another launch.
My second eBook, which was just a rewritten version of the first one, made $16,000 in six weeks. After that, I knew there was something to this whole “make money blogging” thing. Buried in my blog was a business; I just had to find it. Later that year, I released an online course, starting at a low price and gradually raising it with each new class. Every time I launched it, I got more students than the last.
By the end of my second year of blogging, my wife was able to stay home to raise our son, and I was preparing to quit my job. When we did our taxes, we were amazed to see we had not only replaced our income, but tripled it.
What It Takes
Although I’d read all the success stories and heard all the tips, I didn’t realize how much work it would be to build a popular blog.
In my first year of blogging, I wrote over 300 posts for my blog plus 100 guest posts for other websites. To do this, I had to get up every morning at 5:00 a.m. and often stay up well past midnight. I quit most of my other hobbies and focused all my free time on writing.
It wasn’t easy, but I was committed to the dream. Having spent so many years in frustration, unwilling to do the work, I was ready to invest the time — even if it took years — to get the results I wanted.
People often ask me what one thing I did with that made all the difference with my blog. And of course, I can’t think of one. Because it’s a process, a whole hodge-podge of things I did that made it work.
No single strategy can help you to get to your dream. No solitary experience or choice will lead to your big break. Well, except for maybe one:
Don’t give up.
Don’t quit. Keep going. Never stop. Learn from your mistakes, but don’t let go of the dream. You can do this. You will do this — if only you don’t stop.
That’s what’s missing with most blogs and with most writers. Every great story about some legendary entrepreneur I’ve ever read was rife with failure until one hinge moment when it all changed.
What made the difference? Why did Steve Jobs succeeded while thousands others in Silicon Valley didn’t? Why did J.K. Rowling become a worldwide phenomenon while many of her peers never will? And what can ordinary folks like you and me do to live extraordinary lives?
Don’t give up.
If I had to boil it down to three steps, I would say here’s what you need to do:
- Own what you are (i.e. writer, blogger, entrepreneur, etc.).
- Commit to the process. Do uncomfortable things, make friends with influential people, and keep practicing until people notice.
- Keep going. When it’s hard and scary and nobody things you’re any good, don’t give up. Persevere. In the end, we will be telling stories about you, but only if you don’t quit.
So what do you say? Isn’t it time you started really pursuing your dream?
You bet it is.
Jeff Goins is a writer who lives in Nashville. You can follow him on Twitter @JeffGoins and check out his new book, The In-Between.
How I Stopped Waiting to Become a Writer, Quit My Job & Launched My Dream
http://www.problogger.net/archives/2013/08/13/how-i-stopped-waiting-to-become-a-writer-quit-my-job-launched-my-dream/
http://www.problogger.net/archives/category/miscellaneous-blog-tips/feed/
@ProBlogger» Miscellaneous Blog Tips
Blog Tips to Help You Make Money Blogging – ProBlogger
http://www.problogger.net/wp-content/plugins/podpress/images/powered_by_podpress_large.jpg